in this tutorial I will show you how to make a chronometer app with a good UI design, in android studio and java, you will some assets that you will find in this article. you will also learn how to make a custom toggle button using Android Studio, So let's start.
Image assets
first, we need to import some image assets for our project
download these images and add them to your project.

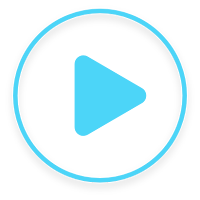
Now let's Do some Code
Style.xml
we want to change the style and make the app without an action bar because we don't need it, and change the status bar color
<resources>
<!-- Base application theme. -->
<style name="AppTheme" parent="Theme.AppCompat.Light.NoActionBar">
<!-- Customize your theme here. -->
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
<!--Now we will change the statut bar color-->
<item name="android:statusBarColor">@color/colorPrimary</item>
</style>
</resources>
Colors.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="colorPrimary">#52dbfe</color>
<color name="colorPrimaryDark">#369ee3</color>
<color name="colorAccent">#D81B60</color>
</resources>
The custom button
we need now to create our custom button.
to do this, right-click on your drawable folder choose new and new drawable file, name it start_pause_btn_bg and add this code
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:state_checked="false"
android:drawable="@drawable/start_button"
/>
<item
android:state_checked="true"
android:drawable="@drawable/pause"
/>
</selector>
The main layout activity
now let's code the main layout of our activity
inside your activity_main.xml file add this code
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<ImageView
android:id="@+id/header"
android:layout_width="match_parent"
android:layout_height="200dp"
android:layout_margin="0dp"
android:src="@drawable/header"
android:scaleType="centerCrop"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<Chronometer
android:id="@+id/chronometer"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="130dp"
app:layout_constraintTop_toTopOf="parent"
android:paddingTop="25dp"
android:textSize="70sp"
android:background="@drawable/bg"
android:textAlignment="center"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
<ToggleButton
android:id="@+id/Toggle"
android:layout_width="150dp"
android:layout_height="150dp"
android:background="@drawable/start_pause_btn_bg"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/chronometer" />
<Button
android:id="@+id/reset_btn"
android:layout_width="90dp"
android:layout_height="90dp"
android:background="@drawable/reset_button"
app:layout_constraintBottom_toBottomOf="@+id/Toggle"
app:layout_constraintStart_toEndOf="@+id/Toggle" />
</androidx.constraintlayout.widget.ConstraintLayout>
now let's add the Java Code
package tn.doctorcode.stopwatch;
import android.os.Bundle;
import android.os.SystemClock;
import android.view.View;
import android.widget.Button;
import android.widget.Chronometer;
import android.widget.CompoundButton;
import android.widget.ToggleButton;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
private Chronometer chronometer;
private long PauseOffSet = 0;
private boolean isPlaying = false;
private ToggleButton toggleButton;
private Button reset_btn;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Let's select our element
chronometer = findViewById(R.id.chronometer);
toggleButton = findViewById(R.id.Toggle);
reset_btn = findViewById(R.id.reset_btn);
// now we need to customize our toggle button
toggleButton.setText(null);
toggleButton.setTextOn(null);
toggleButton.setTextOff(null);
//the event listener will automaticly generated by android studio
toggleButton.setOnCheckedChangeListener(new CompoundButton.OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton compoundButton, boolean b) {
//now let's code our stopwatch
//b is the state of our button, it will be true if we pressed the button otherwise it will be false
if(b){
chronometer.setBase(SystemClock.elapsedRealtime()- PauseOffSet);
chronometer.start();
isPlaying = true;
}else{
chronometer.stop();
PauseOffSet = SystemClock.elapsedRealtime()- chronometer.getBase();
isPlaying = false;
}
}
});
//now we need to add the reset function of our button
// we should check if the chronometre is actually workin to be able to reset otherwise we can't
reset_btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
if(isPlaying){
chronometer.setBase(SystemClock.elapsedRealtime());
PauseOffSet = 0;
chronometer.start();
isPlaying = true;
//now everything should work let's run our app and see it
}
}
});
}
}
Make a Chronometer application In Android Studio and Java
Reviewed by Medics
on
April 02, 2020
Rating:

No comments: